Homework 4 - due Wednesday, 10/14 before class
Remember: All of the programming exercises should be accompanied by an algorithm description, and test cases if the program takes user input or is a function that has parameters and can be called with different values for the parameters.
Submitting this homework: Please try to upload your game onto Blackboard. However, people are continuing to have problems with uploading files onto Blackboard - so, if you get an error message saying that your file type is unacceptable, please send me the game by email and put "CSC 105 Homework 4 Submission" into the subject line. (If Blackboard shows you a message about the submission being successful, you don't have to worry. Your file was successfully uploaded onto Blackboard.)
Drawing Faces
Write a function (for a pygame program) that draws a face. The function should meet this specification:
drawFace(x, y, color)where x is the x-coordinate of the center, y is the y-coordinate of the center, and color is the color.
Demonstrate your function by writing a pygame program that draws several faces in different locations.
Searching for a value in a list
Write a function that checks whether a given value is an element of a given list. The function should return True or False depending on whether the value is an element of the list.
Intersection of two lists
Write a function that takes two lists and returns a list of all values that are elements of both lists.
Recursive Pyramid
Write a recursive function that draws the following picture:
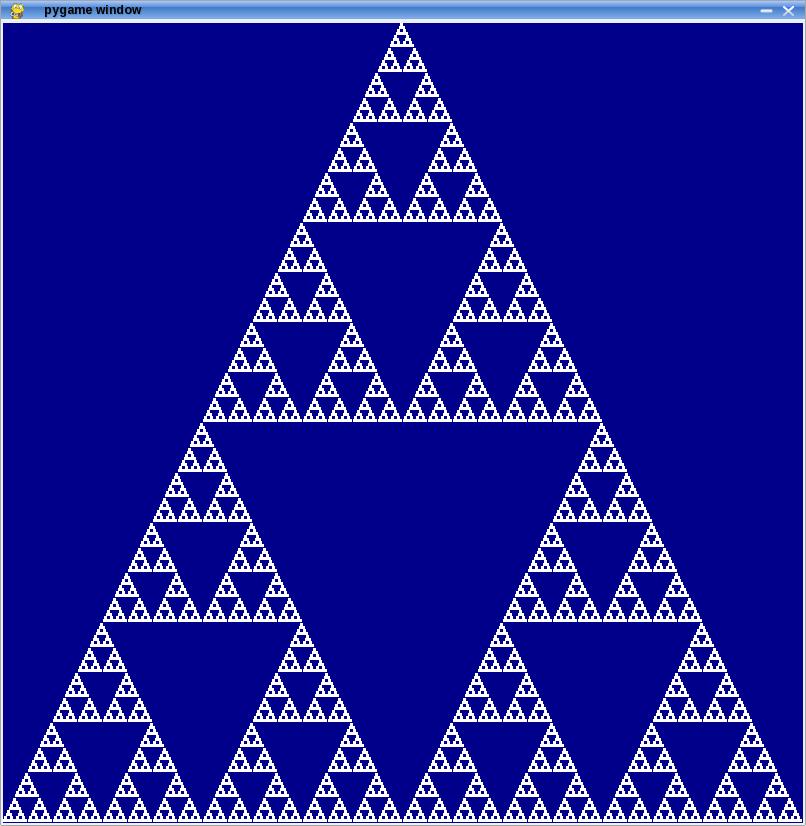
Note that this pyramid is built from square building blocks (not triangular ones even though it may look like it). The following two pictures attempt to show that the structure is based on squares. The picture on the left is a close up of a part of the pyramid, which shows that the base case is just a plain square. The picture on the right shows how the whole structure breaks down into three equal squares and how each of those squares breaks down into three equal squares etc.
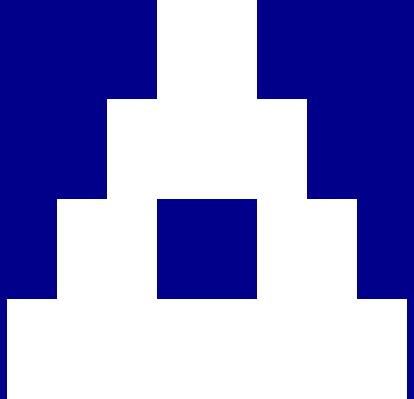
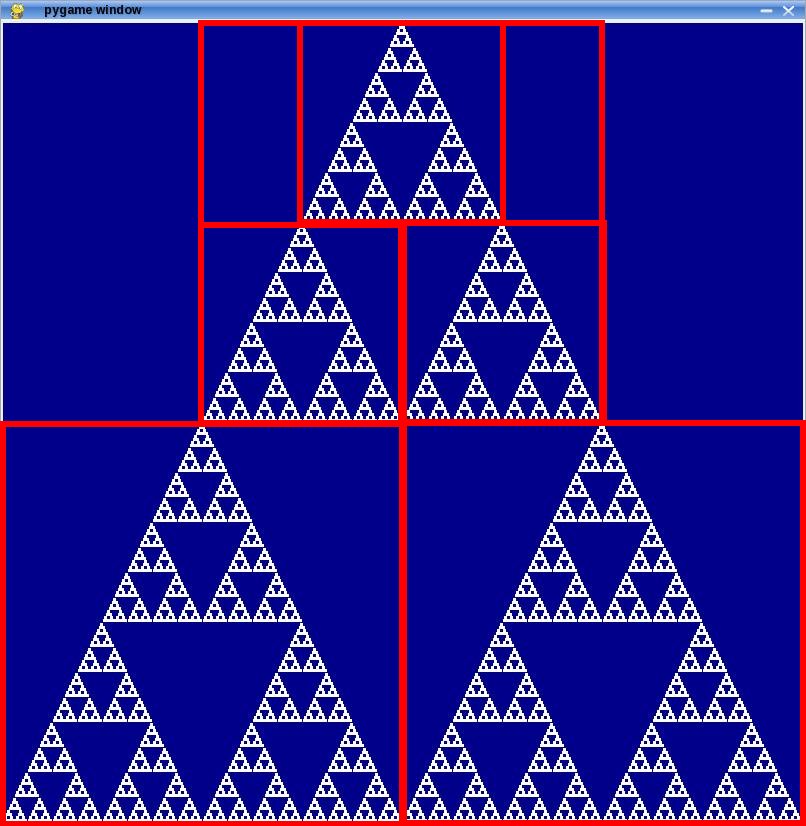
Here are solutions for the two recursive pictures your worked on in class on Thursday: recursive_circles.py, circles_in_circles.py