Homework Week 6 - due Wednesday, May 13, before class
Download this code - it is a working version of what we discussed in class on Thursday. That is, it draws a grid and allows you to change the status of individual cells by clicking on them.
Signaling when to start/stop
Add a boolean variable called go that initially has the value False. This variable will be the sign whether or not we want our system to create new generations.
Now, you need a mechanisms that allows the user to "turn this variable on and off", i.e. to change its value to True and back to False. Here are two possible ways of how to do that; you can choose either one.
1) Select a key (e.g. "s" for start and stop), and code that changes the value of the variable go every time that key is pressed. (This option is easier. See the last homework for how to check for key presses.)
2) Draw a button and change the value of the variable go every time there was a mouse click on the button.
Add a timer
Add a timer variable that sums up the time that is passing.
Add code to the game loop that prints something every 200 milliseconds if the go variable is true. That is, print if the go variable is true and the timer has accumulated 200 milliseconds or more.
Count the life_neighbors
Add a function called count_life_neighbors which takes a grid representation and a row and a column number, and counts the number of cells adjacent to the given cell that are true and returns that number.
Watch out for the edges of the grid! If the given cell is at the edge, it should take the cells at the opposite edge to be adjacent. For example in the following picture, all of the dark red cells are considered to be adjacent to the green cell.
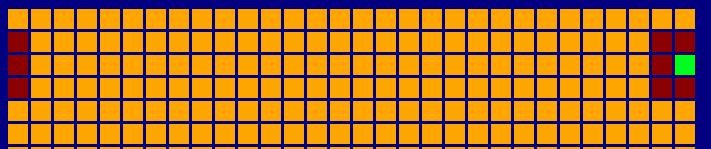
Compute the next generation
Create a function called next_generation that takes a grid and creates a new_grid which represents the next generation. This new grid is returned.
The rules for each cell are as follows:
- Any live cell with fewer than two live neighbours dies, as if by loneliness.
- Any live cell with more than three live neighbours dies, as if by overcrowding.
- Any live cell with two or three live neighbours lives, unchanged, to the next generation.
- Any dead cell with exactly three live neighbours comes to life.
Now, add code to the game loop that calls this function and assigns the return value to the variable grid if the variable go is True and more than 200 milliseconds have passed since the last time the grid was updated.
Have fun trying out some different starting configurations. See the Wikipedia page for inspiration.